Understanding Intersection Type in TypeScript
- Duong Hoang
- Jun 15, 2024
- 1 min read
TypeScript is renowned for its static type system, which empowers developers to define data types with clarity. In this article, we delve into a unique feature of TypeScript: Intersection Type.
What is an Intersection Type in TypeScript?
An Intersection Type in TypeScript allows you to merge multiple types into a single cohesive type. This implies that a variable of an intersection type must meet the requirements of all the types it encompasses. The syntax for Intersection Type is denoted by the & symbol.
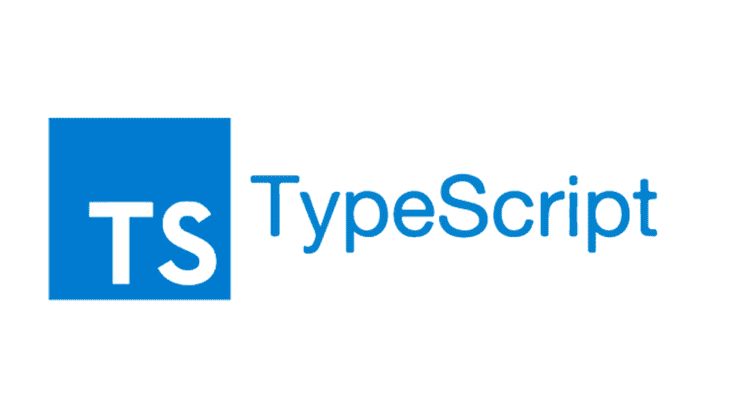
Example of Intersection Type in TypeScript
Consider the following example with two interfaces, Wizard and Chef:
interface Wizard {
magicPower: string;
wand: string;
}
interface Chef {
favoriteDish: string;
kitchenTool: string;
}
We can create an Intersection Type combining both Wizard and Chef like this:
type WizardChef = Wizard & Chef;
Now, the WizardChef type must satisfy both the Wizard and Chef interfaces.
Using Intersection Type in TypeScript
Here’s a practical example of using an Intersection Type in TypeScript:
const wizardChef: WizardChef = {
magicPower: "Fireball",
wand: "Dragon Heartstring",
favoriteDish: "Spaghetti Carbonara",
kitchenTool: "Magic Spatula"
};
In this example, the wizardChef object needs to include all the properties from both Wizard and Chef. If any property is missing, TypeScript will generate an error.
Benefits of Intersection Type in TypeScript
Benefit | Description |
Flexibility | Intersection Type in TypeScript enables you to combine multiple types, creating more complex and versatile types. |
Type Safety | By merging types, you ensure that an object satisfies all the requirements of the combined types, thus reducing runtime errors. |
Code Reusability | Intersection Type in TypeScript helps you reuse defined types, saving time and effort during the development process. |
Conclusion
Intersection Type is a robust feature in TypeScript, enabling developers to create complex data types effortlessly and safely. By understanding and leveraging Intersection Type in TypeScript, you can make the most of its static type system to write high-quality, maintainable code. If you haven't yet explored Intersection Type in TypeScript, start today and experience the benefits it brings to your projects!
Comments