A Comprehensive Comparison Between TypeScript and JavaScript
- Duong Hoang
- Jun 15, 2024
- 6 min read
JavaScript and TypeScript share many similarities, but they also have distinct characteristics that define each language. Personally, I, along with many around me, have gradually transitioned from JavaScript to TypeScript. Why is that? What are the differences between these two languages? And most importantly: which language is better?
In this article, I will compare the differences between these two languages from a programmer's perspective. For regular users, JavaScript is the only option (not that regular users care, right? 😄). Due to the vast amount of knowledge, my understanding is only partial, so I can only compare within that scope xD.
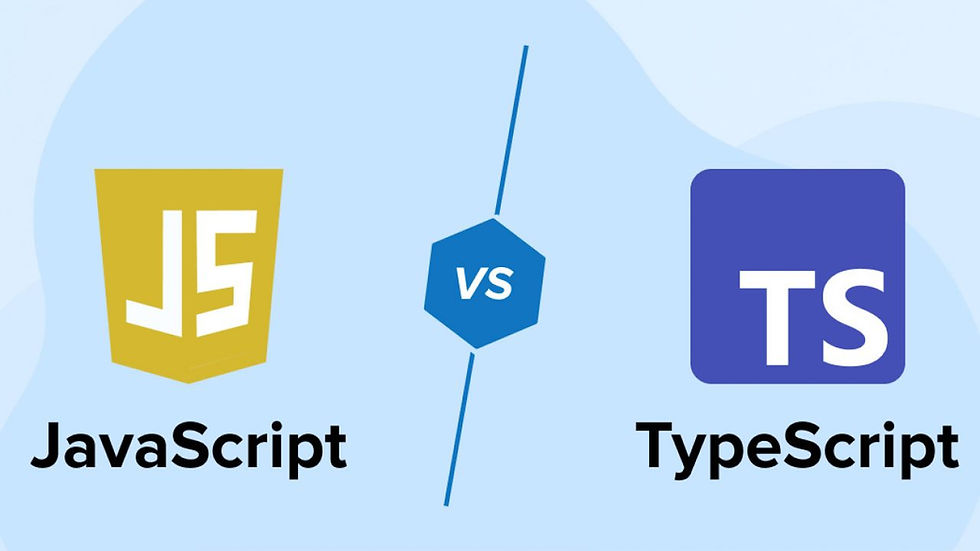
What is JavaScript?
JavaScript (JS) is currently the most popular programming language in the world. It is a high-level programming language that allows for creating flexible and interactive web applications. Along with HTML and CSS, JavaScript is one of the "core" technologies of web applications.
JavaScript is characterized by dynamic typing and the Just-In-Time (JIT) compiler. Additionally, JavaScript supports various programming paradigms, including functional programming, event-driven programming, and OOP. Initially, JavaScript was a client-side language (executed in the browser), but it has evolved to run on the server (via Node.js).
Some notable features of JavaScript include:
The most popular programming language in the world
Cross-platform, multi-paradigm, dynamic typing
Runs on both client and server
JIT compilation
Good compatibility with all browsers
However, working with JavaScript has raised several issues. As JavaScript codebases grow, managing the code becomes more difficult, leading Microsoft to create a different language to address these problems. That language is TypeScript.
What is TypeScript?
As mentioned above, JavaScript was not originally designed to handle large codebases. Working with JavaScript in complex projects can pose various challenges. Hence, TypeScript (TS) was created to tackle these issues.
TypeScript is considered a superset of JavaScript, designed to solve JavaScript problems more efficiently. It adheres to the ECMAScript specification, so its syntax is similar to JavaScript. However, it is better suited for complex projects due to its support for strongly typed variables and error-checking during compilation.
In reality, TypeScript supports both static and dynamic typing. Additionally, it offers many essential features of object-oriented programming, such as inheritance, classes, scopes, namespaces, interfaces, and many other modern features.
TypeScript can be used on both the client and server, just like JavaScript. Another advantage is that JavaScript libraries are also usable with TypeScript.
Some key features of TypeScript include:
Superset of JavaScript, compatible with existing JavaScript libraries
Strongly typed, compiled language, adhering to OOP principles
Easier debugging
Support for static typing
Some Advantages of TypeScript Over JavaScript
TypeScript catches errors during compilation, reducing runtime errors, whereas JavaScript, being an interpreted language, reports syntax errors only at runtime.
TypeScript allows for type-checking during compilation, a feature absent in JavaScript.
TypeScript can be seen as JavaScript + advanced features. The TypeScript compiler can convert TypeScript to any version of JavaScript to run on various browsers.
JavaScript Problems
Fundamentally, TypeScript is a compiled language, meaning it needs to be compiled before execution. Typically, TypeScript is compiled into JavaScript for execution. In the next section, I will discuss the specific differences between these two languages.
Comparing TypeScript and JavaScript
Definitions
Firstly, conceptually, the two languages are vastly different. JavaScript is a scripting language for creating dynamic web pages, whereas TypeScript is a superset of JavaScript with strong typing.
Simply put, TypeScript is JavaScript with additional features that simplify programming, especially those related to data types and managing complex codebases.
However, it should be noted that these modern features are added at the syntactic level during programming and compilation. The end result is that TypeScript is compiled into JavaScript for execution.
Syntax
Both languages adhere to the ECMAScript specification, so their syntax is very similar. For me and many other programmers familiar with JavaScript, transitioning to TypeScript is not difficult. In fact, because the syntax is so similar, if you're lazy, you can just copy JavaScript code into TypeScript, and it will run.
The biggest difference in TypeScript is probably that variables need to have their data types declared (or inferred if there's an initial value). However, I find TypeScript to be backward compatible with JavaScript by providing an "any" data type (used when the data type is unknown or for dynamic typing like JavaScript).
Previously, TypeScript was considered more advanced due to its support for object-oriented programming. However, since ECMAScript 2015, JavaScript has also added many OOP syntaxes (though not as comprehensive as TypeScript). Therefore, in terms of syntax, the two languages do not have significant differences.
Compilation
There is no requirement for compiling JavaScript as it is designed to run directly in the browser (though recently, JavaScript may need to be transpiled to be compatible with older browsers). One issue with this is that many errors are only discovered at runtime.
For example, you might write a function that expects a string parameter, but nothing prevents you or others from passing in an int, float, or even undefined. Some operations (e.g., +, -) work very differently with different data types, making debugging and error fixing in JavaScript more time-consuming.
TypeScript is different; it needs to be compiled and can detect some errors (like in the example above) during this step. This can save programmers a lot of time in finding and fixing errors and can prevent many potential errors from the start. This is significant when working in a team where one often uses others' code.
The downside is that TypeScript must be compiled before it can run, making development and testing more time-consuming, whereas JavaScript can run directly. However, given the benefits mentioned above, the time spent compiling and executing TypeScript is minimal compared to the advantages it brings.
Typing
JavaScript uses dynamic typing, meaning a variable can take any data type (e.g., an integer can later be assigned a string). This is a significant advantage for small scripts (a few lines to a few dozen lines) as it allows for faster programming. However, with large applications having many modules, this can lead to data handling issues because you don't know what type of data a variable holds (or when it was changed).
JavaScript does not support static typing, a programming style where each variable is declared with a data type and will throw an error if assigned a value of a different data type. TypeScript provides this feature.
Static typing is the most crucial feature of TypeScript (perhaps that's why it's named TypeScript). It allows developers to detect data type-related errors during compilation.
Not only the language, but some modern development tools (e.g., VSCode) also have many features to check and detect such errors during coding. Clearly, linting TypeScript code (thanks to static typing) is much easier than JavaScript.
Is JavaScript an OOP Language?
ECMAScript is the language specification, providing syntax, guidelines, and many other descriptions for JavaScript (and TypeScript). Since ECMAScript 2015 (ES6 or ES2015), this specification has added modules, classes, arrow functions, objects, and many other exciting features related to OOP.
Since ES6, JavaScript developers have been able to use classes. However, this feature is not truly object-oriented programming because it operates based on JavaScript's prototype mechanism. Being a prototype-based language (not class-based, though you can code using this keyword), JavaScript is not considered an object-oriented programming language, although it can follow some OOP principles.
Is TypeScript an OOP Language?
The answer to this question is somewhat complicated. Like JavaScript, TypeScript has classes and many related features (namespaces, interfaces, etc.) that allow developers to program in an object-oriented way.
However, TypeScript is compiled into JavaScript for execution (still relying on the prototype mechanism). Moreover, unlike standard object-oriented programming languages (e.g., Java), TypeScript does not require OOP programming. Thus, TypeScript is also often not considered a pure object-oriented language.
In practice, TypeScript, like JavaScript, allows developers to program using various paradigms, such as functional programming or procedural programming.
Example Code in TypeScript and JavaScript
JavaScript
const mars = {
name: "Mars",
gravity: 3.721
};
mars.mass; // undefined
function weight(mass, gravity) {
return mass * gravity;
}
TypeScript
// This code is similar to `let destination: string` but not needed as TypeScript infers the data type
let destination = 'Mars';
Is TypeScript Better Than JavaScript?
JavaScript has risen to become the most popular programming language in the world for many years, while TypeScript has only become popular since 2017. According to StackOverflow surveys, JavaScript remains the most popular language, but TypeScript is also rapidly gaining traction.
After comparing, most programmers agree that TypeScript has more modern features than JavaScript. But is it really better?
Fairly speaking, any tool is best when used for the most appropriate task. For small projects, TypeScript is not really necessary. In such cases, JavaScript is better due to its good compatibility with browsers and its lightweight nature (runs immediately without needing compilation or configuration). For example, my blog is just a static page and doesn't require much code to run, so JavaScript is the right choice 👍.
Compared to JavaScript, compatibility with browsers is a major drawback of TypeScript.
Комментарии