Removing Elements from an Array in JavaScript
- Duong Hoang
- Jul 14, 2024
- 3 min read
Removing elements from an array in JavaScript is a common problem that most developers encounter. JavaScript offers numerous solutions to address this problem, each with its own advantages and disadvantages, suitable for specific use cases. This article will summarize various methods to remove one or more elements from an array in JavaScript.
Broadly, the methods can be categorized into two types:
Mutable ways: These methods modify the original array.
Immutable ways: These methods do not modify the original array.
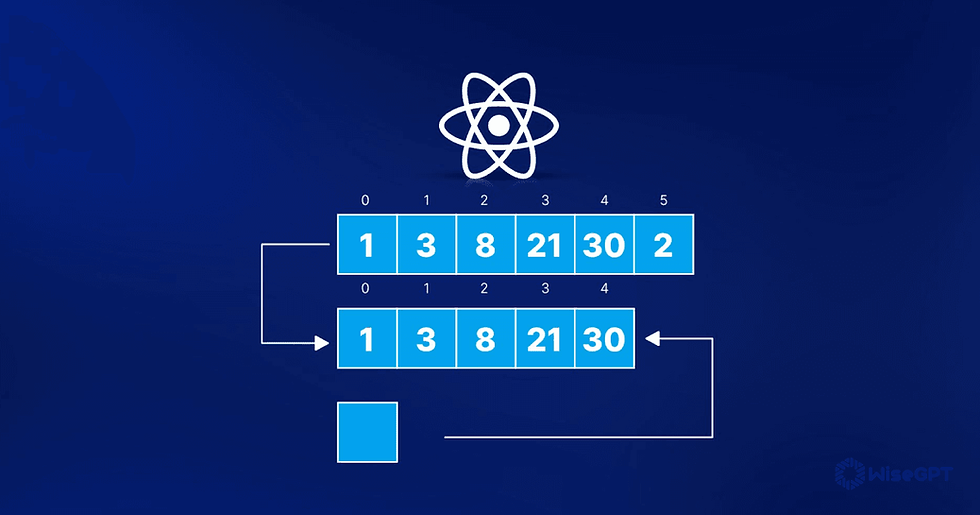
Mutable Ways
Removing the Last Element with pop()
The pop() method removes the last element from the array and returns its value. If the array is empty, it returns undefined.
Example:
const arr = ["a", "b", "c", "d"];
console.log(arr); // => (4) ["a", "b", "c", "d"]
const item = arr.pop();
console.log(arr); // => (3) ["a", "b", "c"]
console.log(item); // => d
Removing the First Element with shift()
The shift() method removes the first element from the array and returns its value. If the array is empty, it returns undefined.
Example:
const arr = ["a", "b", "c", "d"];
console.log(arr); // => (4) ["a", "b", "c", "d"]
const item = arr.shift();
console.log(arr); // => (3) ["b", "c", "d"]
console.log(item); // => a
Removing One or More Elements at a Specific Position with splice()
The splice() method can remove one or more elements from an array. Additionally, it can add elements to the array, but in this article, we'll focus on removing elements.
Syntax for using splice() to remove elements from a JavaScript array is:
array.splice(start, n);
Where:
start: The index at which to start removing elements.
n: The number of elements to remove.
The method returns the array of removed elements.
Example:
const arr = ['a', 'b', 'c', 'd'];
console.log(arr); // => (4) ["a", "b", "c", "d"]
const removed = arr.splice(1, 2);
console.log(arr); // => (2) ["a", "d"]
console.log(removed); // => (2) ["b", "c"]
In this code, the statement arr.splice(1, 2) removes elements starting from index 1 (which is 'b'), and the number of elements removed is 2. Therefore, the removed elements are 'b' and 'c', and the original array becomes ["a", "d"].
Immutable Ways
Removing Elements by Index with slice() and concat()
To remove an element at index i from array arr, you can use the slice() method twice to copy from the original array into two new sub-arrays (without changing the original array).
First, copy elements from position 0 to position i - 1. Second, copy elements from position i + 1 to the last element. Then use the concat() method to merge these two sub-arrays into a single array.
Example:
const arr = ["a", "b", "c", "d", "e"];
console.log(arr); // => (5) ["a", "b", "c", "d", "e"]
const i = 2;
const a1 = arr.slice(0, i);
const a2 = arr.slice(i + 1, arr.length);
const new_arr = a1.concat(a2);
console.log(arr); // => (5) ["a", "b", "c", "d", "e"]
console.log(new_arr); // => (4) ["a", "b", "d", "e"]
You can also use the spread operator to merge two arrays:
const new_arr = [...a1, ...a2];
The above example removes one element from the array, but you can apply it to remove multiple elements based on index and quantity.
Example:
const arr = ["a", "b", "c", "d", "e"];
console.log(arr); // => (5) ["a", "b", "c", "d", "e"]
const i = 1;
const amount = 2;
const a1 = arr.slice(0, i);
const a2 = arr.slice(i + amount, arr.length);
const new_arr = a1.concat(a2);
console.log(arr); // => (5) ["a", "b", "c", "d", "e"]
console.log(new_arr); // => (3) ["a", "d", "e"]
In this code, two elements starting from index 1 are removed from the array, which are 'b' and 'c'.
Removing Elements by Value with filter()
If you know the value of the element to be removed from the array, you can use the filter() method. This method creates a new array with all elements that pass the test implemented by the provided function.
Example:
const arr = ["a", "b", "c", "d", "e"];
console.log(arr); // => (5) ["a", "b", "c", "d", "e"]
const valueToRemove = "b";
const new_arr = arr.filter(item => item !== valueToRemove);
console.log(arr); // => (5) ["a", "b", "c", "d", "e"]
console.log(new_arr); // => (4) ["a", "c", "d", "e"]
This code creates a new array including all elements that are different from the value to be removed, which is 'b'. Naturally, you can use this method to remove multiple elements.
Example:
const arr = ["a", "b", "c", "d", "e"];
console.log(arr); // => (5) ["a", "b", "c", "d", "e"]
const valuesToRemove = ["b", "e"];
const new_arr = arr.filter(item => !valuesToRemove.includes(item));
console.log(arr); // => (5) ["a", "b", "c", "d", "e"]
console.log(new_arr); // => (3) ["a", "c", "d"]
In this code, all elements with values 'b' and 'e' are removed from the array, resulting in the new array ["a", "c", "d"].
Commentaires